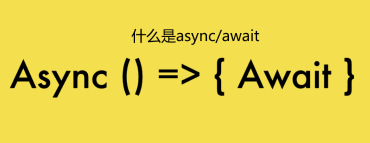
关于async/await的使用方法
什么是async/await?
async/await是JavaScript中处理异步操作的一种语法糖,基于Promise。它让异步代码看起来像同步代码,更易读、更易维护。
- async:声明一个函数是异步的,总是返回一个Promise,即使内部没用await。
- await:暂停异步函数的执行,等待Promise解析(resolve)或拒绝(reject),只能在async函数内部使用。
async/await和Promise的区别?
async/await主要用于处理异步操作,为了直观对比,代码展示下有无async/await的区别
- 使用Promise的链式执行
function getUser() {
return fetch('https://api.example.com/user').then(res => res.json());
}
function getPosts(userId) {
return fetch(`https://api.example.com/posts/${userId}`).then(res => res.json());
}
getUser()
.then(user => {
console.log("用户:", user);
return getPosts(user.id);
})
.then(posts => {
console.log("文章:", posts);
})
.catch(error => {
console.log("错误:", error);
});
- 使用async/await
async function postMsg() {
try {
const user = await fetch('https://api.example.com/user').then(res => res.json());
console.log("用户:", user);
const posts = await fetch(`https://api.example.com/posts/${user.id}`).then(res => res.json());
console.log("文章:", posts);
} catch (error) {
console.log("错误:", error);
}
}
postMsg();
什么时候用到async/await?
需要处理异步操作(网络请求、文件读写、定时任务等)
当你不使用async/await时,异步操作(如fetch)不会阻塞代码执行,后续代码会立即运行,而异步操作的结果会在未来某个时间点返回。这是因为JavaScript是单线程的,异步操作(如网络请求)会被放到事件循环中,等主线程空闲时处理。
console.log(1);
fetch('https://api.example.com/data') // 模拟异步请求,返回结果假设是2
.then(response => response.json())
.then(data => console.log(data)); // 打印 2
console.log(3);
输出结果
1
3
2
- console.log(1):立即执行,打印1。
- fetch():发起异步请求,但不等待结果,主线程继续往下走。
- console.log(3):立即执行,打印3。
- fetch的.then():等到网络请求完成(比如几百毫秒后),打印2。
async function run() {
console.log(1);
const response = await fetch('https://api.example.com/data'); // 假设返回 2
const data = await response.json();
console.log(data);
console.log(3);
}
run();
输出结果
1
2
3
- console.log(1):立即执行,打印1。
- await fetch():暂停run函数,等待请求完成,拿到结果(假设是2)。
- await response.json():继续等待解析JSON,得到data。
- console.log(data):打印2。
- console.log(3):打印3。
如果多个异步操作互相独立,用Promise.all并行执行:
async function fetchBoth() {
const [user, posts] = await Promise.all([
fetch('user'),
fetch('posts')
]);
}
目录